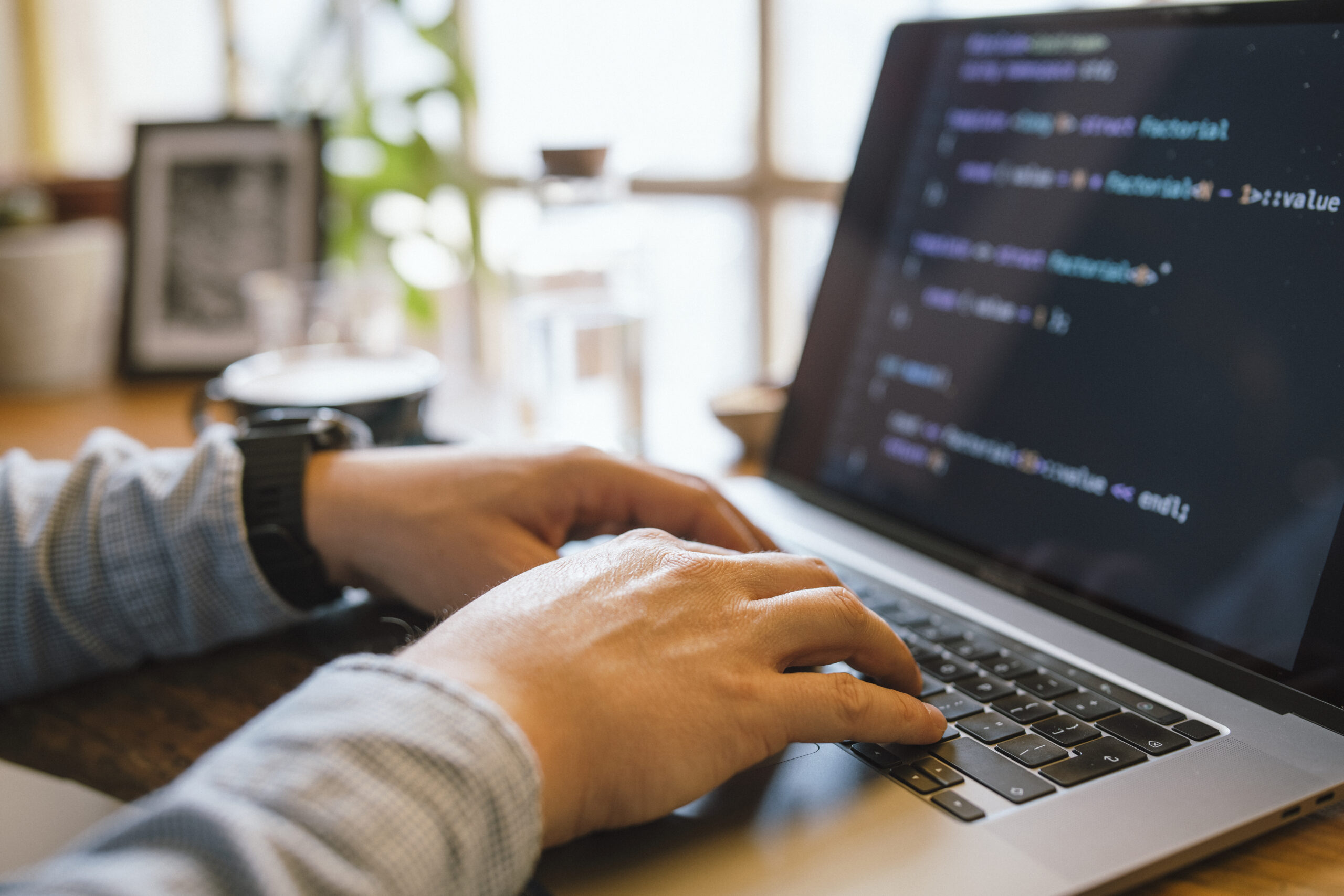
Debugging is The most vital — yet frequently disregarded — techniques inside of a developer’s toolkit. It's not just about fixing broken code; it’s about comprehending how and why items go Mistaken, and Finding out to Assume methodically to unravel challenges successfully. Irrespective of whether you are a starter or simply a seasoned developer, sharpening your debugging competencies can help you save several hours of annoyance and considerably transform your productiveness. Here's many approaches to aid developers level up their debugging activity by me, Gustavo Woltmann.
Learn Your Equipment
One of several quickest ways builders can elevate their debugging capabilities is by mastering the equipment they use each day. While crafting code is just one Section of advancement, knowing ways to communicate with it efficiently during execution is Similarly crucial. Contemporary enhancement environments appear equipped with impressive debugging capabilities — but many builders only scratch the surface of what these equipment can do.
Take, such as, an Integrated Development Ecosystem (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These tools assist you to set breakpoints, inspect the worth of variables at runtime, phase via code line by line, and perhaps modify code about the fly. When utilized the right way, they Allow you to notice specifically how your code behaves all through execution, that's invaluable for tracking down elusive bugs.
Browser developer tools, for example Chrome DevTools, are indispensable for entrance-stop builders. They permit you to inspect the DOM, watch community requests, check out authentic-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and network tabs can switch frustrating UI concerns into workable responsibilities.
For backend or method-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB supply deep Management around operating processes and memory administration. Discovering these tools could have a steeper Mastering curve but pays off when debugging performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into snug with version Manage programs like Git to be familiar with code history, discover the exact minute bugs ended up released, and isolate problematic changes.
Ultimately, mastering your equipment usually means going beyond default settings and shortcuts — it’s about creating an intimate knowledge of your advancement surroundings to ensure when troubles occur, you’re not missing in the dead of night. The higher you already know your applications, the greater time you may shell out fixing the actual difficulty as an alternative to fumbling by means of the method.
Reproduce the challenge
The most vital — and often ignored — actions in efficient debugging is reproducing the issue. Before leaping in the code or generating guesses, developers need to produce a regular setting or situation exactly where the bug reliably appears. Without the need of reproducibility, repairing a bug turns into a game of prospect, typically leading to squandered time and fragile code alterations.
The first step in reproducing a dilemma is collecting as much context as is possible. Request questions like: What steps led to The difficulty? Which surroundings was it in — development, staging, or generation? Are there any logs, screenshots, or error messages? The greater detail you may have, the simpler it results in being to isolate the exact disorders beneath which the bug takes place.
As soon as you’ve gathered more than enough details, try to recreate the challenge in your neighborhood surroundings. This may suggest inputting a similar info, simulating identical user interactions, or mimicking technique states. If the issue appears intermittently, look at writing automated checks that replicate the edge circumstances or point out transitions involved. These exams not simply help expose the trouble but will also stop regressions Later on.
In some cases, the issue could be natural environment-specific — it might come about only on sure functioning methods, browsers, or beneath unique configurations. Using resources like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating these types of bugs.
Reproducing the issue isn’t only a phase — it’s a way of thinking. It necessitates tolerance, observation, and a methodical method. But after you can persistently recreate the bug, you happen to be by now midway to repairing it. That has a reproducible state of affairs, You may use your debugging applications more effectively, test potential fixes safely, and communicate more clearly with your workforce or buyers. It turns an summary criticism right into a concrete problem — and that’s where builders thrive.
Browse and Have an understanding of the Mistake Messages
Mistake messages are frequently the most precious clues a developer has when one thing goes Incorrect. Instead of seeing them as disheartening interruptions, builders really should understand to deal with error messages as immediate communications through the program. They frequently show you just what exactly took place, exactly where it transpired, and from time to time even why it occurred — if you know how to interpret them.
Get started by looking through the message carefully As well as in complete. Many builders, especially when less than time strain, glance at the 1st line and right away start building assumptions. But deeper from the error stack or logs may perhaps lie the real root trigger. Don’t just duplicate and paste error messages into search engines like google and yahoo — read through and understand them initially.
Break the mistake down into parts. Could it be a syntax mistake, a runtime exception, or possibly a logic mistake? Does it place to a specific file and line range? What module or perform activated it? These questions can information your investigation and point you toward the liable code.
It’s also useful to be aware of the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java frequently observe predictable patterns, and Understanding to acknowledge these can drastically accelerate your debugging course of action.
Some errors are obscure or generic, As well as in These situations, it’s very important to examine the context through which the mistake happened. Verify relevant log entries, enter values, and up to date changes inside the codebase.
Don’t forget compiler or linter warnings possibly. These often precede greater troubles and supply hints about opportunity bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Finding out to interpret them the right way turns chaos into clarity, helping you pinpoint problems more quickly, lessen debugging time, and turn into a additional economical and self-assured developer.
Use Logging Sensibly
Logging is one of the most potent resources within a developer’s debugging toolkit. When employed properly, it offers true-time insights into how an application behaves, aiding you recognize what’s occurring beneath the hood with no need to pause execution or stage with the code line by line.
An excellent logging method begins with understanding what to log and at what level. Common logging levels involve DEBUG, Facts, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information all through enhancement, Details for standard activities (like productive begin-ups), Alert for probable troubles that don’t break the application, Mistake for true issues, and Lethal if the program can’t carry on.
Stay clear of flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure vital messages and decelerate your method. Focus on critical activities, state improvements, input/output values, and important determination points in your code.
Structure your log messages clearly and continually. Contain context, such as timestamps, request IDs, and performance names, so it’s simpler to trace issues in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
For the duration of debugging, logs let you observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all without halting This system. They’re especially worthwhile in production environments in which stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about balance and clarity. That has a well-imagined-out logging tactic, you are able to decrease the time it will require to spot concerns, get further visibility into your applications, and Enhance the Over-all maintainability and trustworthiness of your code.
Feel Just like a Detective
Debugging is not merely a technical activity—it's a sort of investigation. To correctly determine and resolve bugs, builders ought to solution the process like a detective solving a thriller. This frame of mind can help stop working elaborate issues into manageable components and stick to clues logically to uncover the basis lead to.
Start out by accumulating proof. Think about the indications of the problem: mistake messages, incorrect output, or performance problems. Much like a detective surveys a crime scene, collect as much relevant info as you are able to with out jumping to conclusions. Use logs, test cases, and person experiences to piece alongside one another a transparent photo of what’s taking place.
Up coming, type hypotheses. Inquire your self: What could possibly be producing this actions? Have any improvements not long ago been manufactured for the codebase? Has this problem occurred right before underneath related situations? The objective is to slender down opportunities and recognize potential culprits.
Then, exam your theories systematically. Endeavor to recreate the trouble inside a managed setting. In the event you suspect a selected purpose or component, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, ask your code issues and Allow the outcomes guide you closer to the reality.
Fork out close notice to modest particulars. Bugs normally cover within the the very least anticipated sites—just like a lacking semicolon, an off-by-one particular error, or maybe a race situation. Be complete and affected person, resisting the urge to patch The difficulty without having totally being familiar with it. Short term fixes may cover the actual difficulty, just for it to resurface later.
And finally, keep notes on Whatever you tried using and discovered. Equally as detectives log their investigations, documenting your debugging process can preserve time for upcoming concerns and enable Other people recognize your reasoning.
By thinking like a detective, developers can sharpen their analytical competencies, method challenges methodically, and become simpler at uncovering concealed challenges in complex techniques.
Produce Exams
Composing assessments is among the simplest methods to increase your debugging competencies and All round growth performance. Checks not only aid catch bugs early but will also function a safety net that gives you self-assurance when generating improvements towards your codebase. A well-tested application is easier to debug because it permits you to pinpoint specifically the place and when a challenge takes place.
Get started with device assessments, which center on particular person capabilities or modules. These smaller, isolated assessments can speedily expose no matter if a selected bit of logic is Doing work as predicted. Every time a take a look at fails, you quickly know wherever to seem, drastically minimizing time invested debugging. Unit tests are Primarily practical for catching regression bugs—challenges that reappear just after Beforehand staying fastened.
Following, integrate integration tests and end-to-end checks into your workflow. These enable make sure many portions of your software perform together effortlessly. They’re notably valuable for catching bugs that happen in complex devices with a number of components or products and services interacting. If a thing breaks, your exams can show you which Component of the pipeline failed and less than what problems.
Creating checks also forces you to Believe critically regarding your code. To test a feature adequately, you'll need to be familiar with its inputs, anticipated outputs, and edge cases. This standard of understanding Obviously prospects to raised code construction and less bugs.
When debugging an issue, composing a failing test that reproduces the bug might be a robust first step. When the test fails persistently, you can target correcting the bug and observe your take a look at pass when The problem is fixed. This method makes sure that a similar bug doesn’t return in the future.
In a nutshell, writing exams turns debugging from the disheartening guessing sport into a structured and predictable course of action—helping you catch a lot more bugs, speedier and more reliably.
Get Breaks
When debugging a difficult challenge, it’s easy to become immersed in the trouble—watching your display screen for hrs, seeking solution following Developers blog Remedy. But Among the most underrated debugging applications is solely stepping absent. Using breaks will help you reset your brain, lower annoyance, and infrequently see The difficulty from the new standpoint.
If you're much too close to the code for as well extended, cognitive tiredness sets in. You could commence overlooking apparent mistakes or misreading code that you simply wrote just hours earlier. In this point out, your Mind gets considerably less productive at difficulty-solving. A brief stroll, a coffee break, or even switching to a different endeavor for ten–quarter-hour can refresh your target. Numerous developers report getting the foundation of a challenge once they've taken time to disconnect, permitting their subconscious get the job done while in the track record.
Breaks also assistance protect against burnout, Specially throughout longer debugging classes. Sitting before a display screen, mentally stuck, is don't just unproductive but in addition draining. Stepping away helps you to return with renewed Strength as well as a clearer mindset. You may perhaps out of the blue discover a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
When you’re stuck, a good general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–ten minute crack. Use that time to maneuver about, extend, or do some thing unrelated to code. It could really feel counterintuitive, Primarily below limited deadlines, however it essentially contributes to a lot quicker and simpler debugging in the long run.
In a nutshell, taking breaks just isn't an indication of weakness—it’s a wise tactic. It gives your brain Place to breathe, improves your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Every bug you come across is much more than simply a temporary setback—It really is a chance to mature as a developer. No matter whether it’s a syntax mistake, a logic flaw, or simply a deep architectural issue, each one can educate you anything important if you make an effort to mirror and examine what went Erroneous.
Get started by inquiring yourself a couple of crucial queries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with better practices like device tests, code reviews, or logging? The responses often expose blind places in the workflow or being familiar with and help you build more powerful coding behavior shifting forward.
Documenting bugs can also be an excellent habit. Continue to keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, how you solved them, and Everything you discovered. As time passes, you’ll begin to see styles—recurring troubles or frequent errors—that you could proactively avoid.
In workforce environments, sharing That which you've uncovered from a bug with your friends could be Particularly powerful. Irrespective of whether it’s by way of a Slack message, a brief publish-up, or a quick awareness-sharing session, supporting Other individuals avoid the similar concern boosts team effectiveness and cultivates a stronger Discovering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as necessary elements of your development journey. In spite of everything, a number of the most effective developers are usually not the ones who generate excellent code, but individuals that continually master from their blunders.
Eventually, Each and every bug you take care of adds a different layer for your ability established. So up coming time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, much more able developer thanks to it.
Conclusion
Increasing your debugging skills normally takes time, practice, and persistence — although the payoff is large. It tends to make you a far more economical, confident, and capable developer. The subsequent time you happen to be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be improved at Everything you do.